A random fractal
(10 pts)
Respond to this question to answer the random fractal question that appears under assignments for Monday, April 13 on our online presentations page. Be sure to include:
- The question itself,
- The code you used to define the IFS, and
- An image of your fractal
Input
You should input your IFS in the code box in the upper left. Most of the examples show how to use some built in functions (like scale
and rotate
) to specify affine functions. You folks should probably be more comfortable specifying each function as a pair [M,v]
, where M
is a matrix and v
is a shift vector. The "Barnsley fern" example in the app is specified that way. Also, try the following code that should generate a square:
M = [[1/2,0],[0,1/2]]; [ [M,[-1,-1]], [M,[1,-1]], [M,[1,1]], [M,[-1,1]] ]
Your input should look something like that, though you'll have a different scale factor and a fifth function with rotation.
Comments
My random fractal required that it consists of 4 pieces scaled by the factor 0.26
and one piece scaled by the factor 0.55 and rotated through the angle 3.16 radians.
My first matrix is only responsible for scaling the input by 0.26 so it'll defined as $
M =
\begin{bmatrix}
0.26 & 0 \\
0 & 0.26
\end{bmatrix} $. For the second matrix I right multiplied the general 2D rotation matrix of 3.16 radians, which is written as $
R =
\begin{bmatrix}
cos(3.16) & -sin(3.16) \\
sin(3.16) & cos(3.16)
\end{bmatrix} $, on the scalar matrix $
M =
\begin{bmatrix}
0.55 & 0 \\
0 & 0.55
\end{bmatrix}
$. I named the resulting matrix $N$. The initial vectors of the IFS are the centers of the squares in the first fractal image.
My python code for this is
Here is the image from the IFS visualizer.
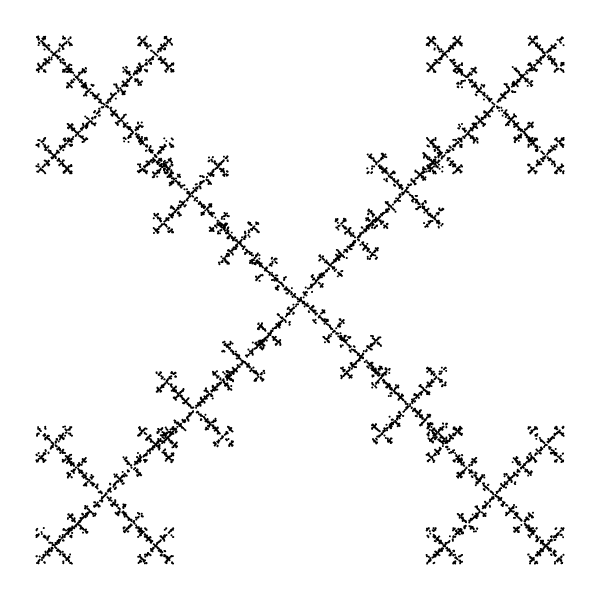
The following was my assignment:
"Your mission is to find an IFS to generate a fractal that consists of 4 pieces scaled by the factor 0.12 and one piece scaled by the factor 0.77 and rotated through the angle 0.48 radians. Your fractal should have some symmetry so that a first approximation looks like so:"
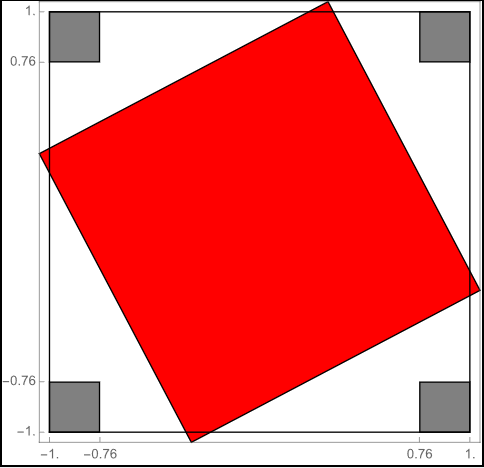
Using the IFS Visualizer, I used a deterministic algorithm with a tolerance of 0.02 and depth of 1. The following was my initial seed:
To generate the scaled and rotated squares, I used the following code:
The result is the following plot, which matches the original:
Using a depth of 20, the fractal that arises is the following:
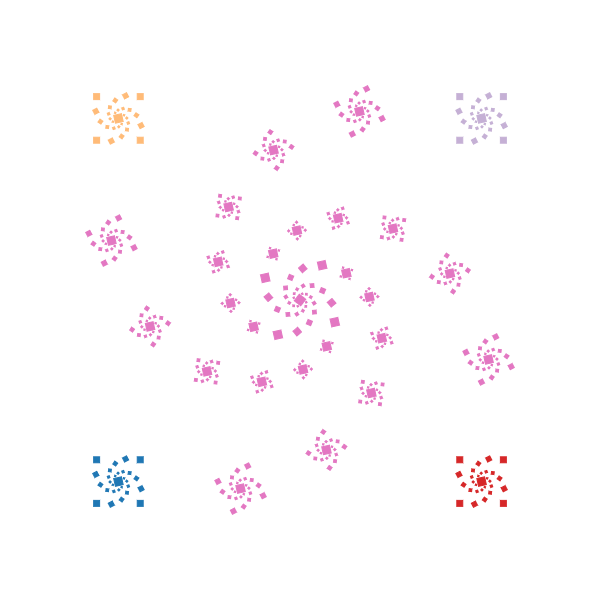
@joshuam and @PrinceHumperdinck Your work looks great so far - you're almost there! The idea now is to generate the fractal that arises. For example, I could say that the following illustrates the effect of the Sierpinski IFS on a triangle:
But the fractal that arises is more complex:
Note that I used the same IFS to generate both pictures but with different settings. The first picture illustrates one step of the deterministic algorithm, as you two did. The second picture uses the random algorithm.
@PrinceHumperdinck Great!!
I had one mission, to classify the fractal responsible for the rotation of one LARGE square by 3.53 rads, and the scaling of this same square by .62. It would be at the center of it all. Surrounded by its 4 amigos (friends in spanish guys..) , scaled by .21 and showing no signs of a rotation.
I loaded in some useful tools
I defined my matrices as so:
The matrix applied to the center piece to achieve rotation was
$R=\left[\begin{matrix}
cos(3.53) & -sin(3.53) \\
sin(3.53) & cos(3.53)\\
\end{matrix}\right]$
The matrix responsible for scaling this same square, the LEADER:
$S_1 = \left[\begin{matrix}
0.62 & 0 \\
0 & 0.62\\
\end{matrix}\right]$
Finally, for the followers, the sheeple, they hadnt been rotated, only scaled. This scaling was expressed as:
$S_2 = \left[\begin{matrix}
0.21 & 0\\
0 & 0.21 \\
\end{matrix}\right]$
Using these matrices, I was able to compose a matrix that described the 2 separate scenarios of squares.
I used IFS to set the initial seed as:
and the scaling of
So I did this with multiple values for "depth" which I will upload. I left my tolerance as .02.
For a depth of 1:
For a depth of 3:
and finally, a depth of 8, where you see the fractal blossom into its truest form:
This be it.
My question:
"Your mission is to find an IFS to generate a fractal that consists of 4 pieces scaled by the factor 0.38 and one piece scaled by the factor 0.38 and rotated through the angle 3.34 radians. Your fractal should have some symmetry so that a first approximation looks like so:
Using the IFS Visualizer, I set the seed to
and manipulated the elements using the following code
With a tolerance of 0.02 and a depth of 1 as the settings of the deterministic algorithm, I plotted the result of the first approximation.
Switching the algorithm to random, I plotted the resulting fractal.
Yo.
Ya know the dealio:
1.) Go to Mark's online IFS Visualizer App
2.) Click Deterministic
3.) If ya got a square, like I did, set your seed too:
[
[-1,-1],[1,-1],[1,1],[-1,1]
]
note: the initial image is a block of just area =1. thats where the scaling and placement comes in.
4.) Pay attention to the scaling and rotation given in your problem and input them into the appropriate place.
mine was: "Your mission is to find an IFS to generate a fractal that consists of 4 pieces scaled by the factor 0.2 and one piece scaled by the factor 0.64 and rotated through the angle 0.57 radians. Your fractal should have some symmetry so that a first approximation looks like so:"
So my tiny pieces had a scaling of .2, bigger one had a scaling of .64, and the bigger one was turned .57 rads. In the programs input, that language looks like:
Putting this into the IFS Visualizer looks like:
[ scale(0.2, [-1,-1]),
scale(0.2, [1,-1]),
scale(0.2, [1,1]),
scale(0.2, [-1,1]),
scale(0.64).compose(rotate(.57))
]
The first iteration looks like so:
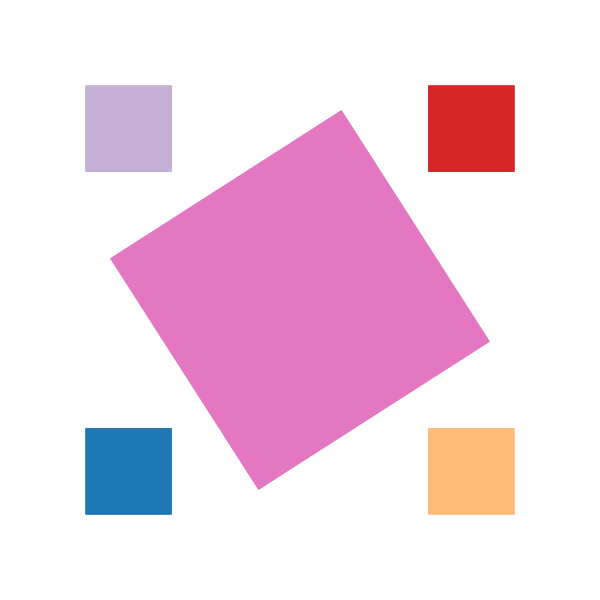
Second iteration looks like:
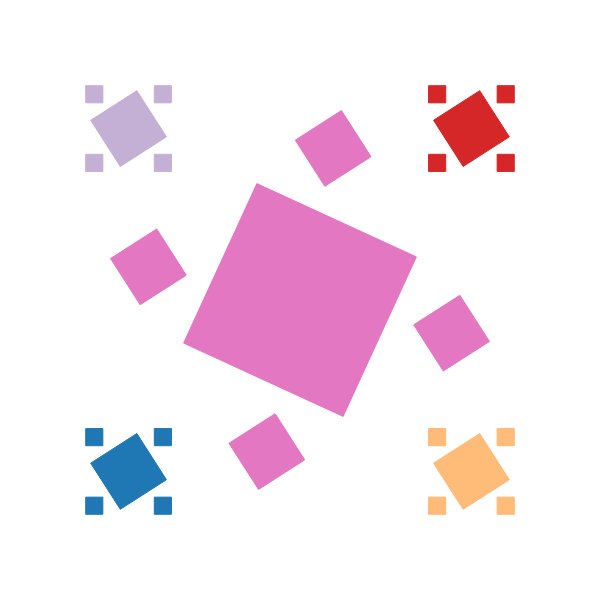
Its the Final Form!
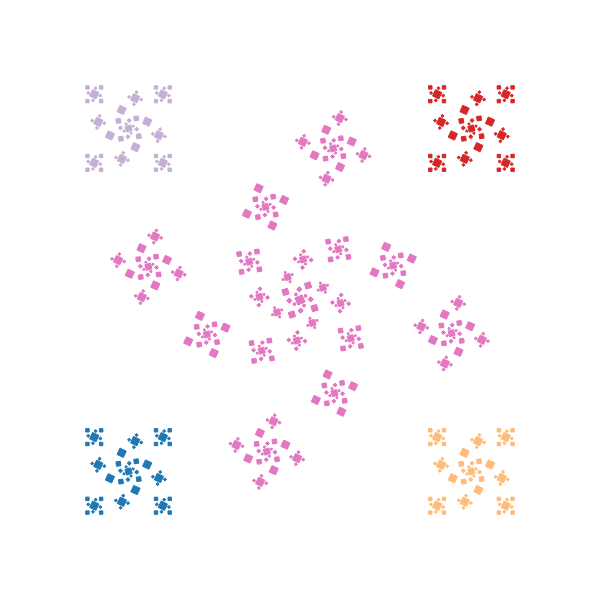
Don't really have much to contribute on the assignment, that hasn't been done before me..So I thought I'd share some fun stuff here:
https://electricsheep.org/
Cool screen saver IFS system generator/open source/ cloud powered thing.
Another fun fact: IFS are used to generate things in video games, such as grass and background objects. It takes less processing power to code an IFS to render, than put in a slew of data files for grass.
I remember hearing, in an interview with the film crew for "The Two Towers," that they used IFS in the CGI for the battle of Helm's Deep, but I could find that online tonight. Anyone else hear about that or am I imagining things?
My goal for this problem is to generate a fractal that consists of 4 pieces scaled by the factor 0.16 and one piece scaled by the factor 0.71 and rotated through the angle 3.61 radians.
In order to generate this fractal, I first input the initial seed
I then added the following IFS to the visualizer:
Looking at this deterministically, with a depth of 1 and tolerance of .02, we get the following image:
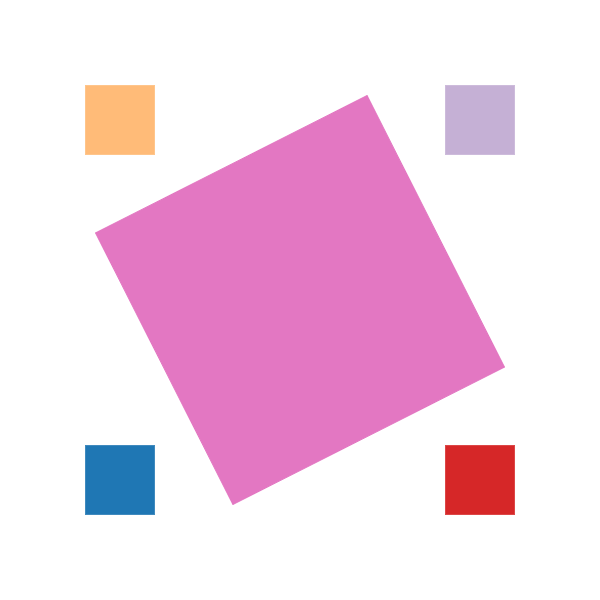
To get the general idea of the fractal, I looked at this with random points, setting it to generate 250000 points:
My mission was to generate a fractal which contained four pieces scaled by 0.29, along with one piece scaled by 0.5 and rotated by 5.43 radians.
Using the IFS Visualizer, the following was my seed:
The following code was used to generate my fractal:
Using a depth of 1, I generated the following fractal.
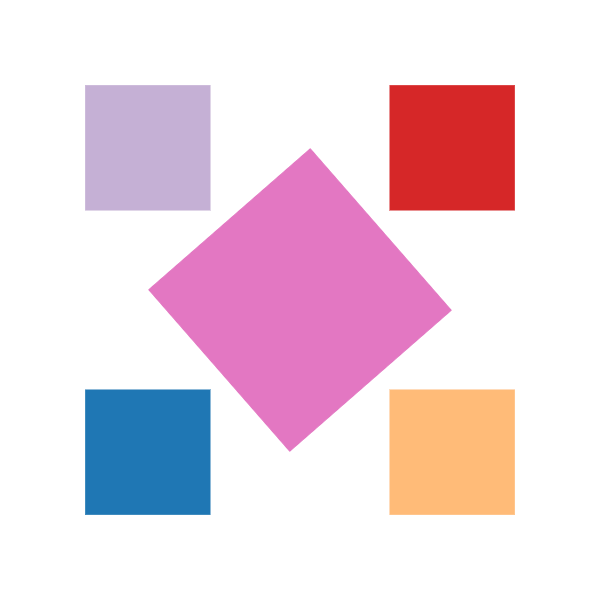
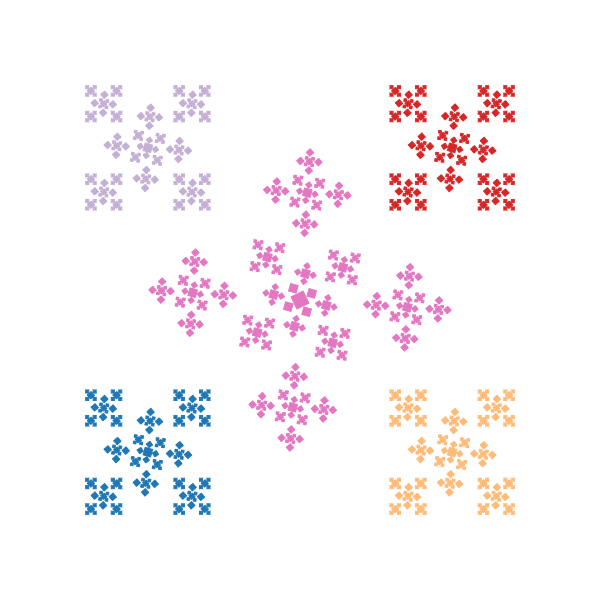
Then, using a depth of 5 resulted in this fractal:
My assignment was to find an IFS that generates a fractal consisting of 4 pieces scaled by the factor 0.37 and one piece scaled by the factor 0.40 and rotated through the angle 4.16 radians.
After observing the Koch Curve example I floundered around with the formatting until I realized everybody else here had already figure it out... Thus, my final IFS code took the form:
Using a deterministic initial seed of
the first iteration looked like
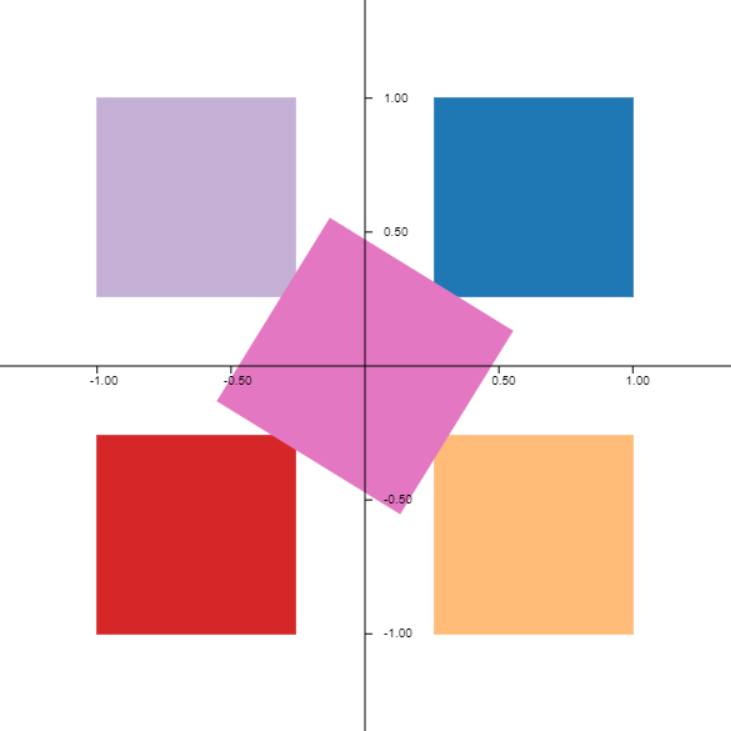
which matched the figure generated for my assignment.
After 19 more iterations, my IFS yielded the fractal below.
My assignment was to find an IFS for the following fractal:
where each of the four smaller pieces are scaled by 0.31 and the larger central piece is scaled by 0.48 and rotated by 1.68 rad.
The IFS consists of 5 functions, one for each piece. My input for the IFS visualizer was:
With the algorithm set to random and the number of points set to 100000 the generated fractal was:
The following was my assignment:
"Your mission is to find an IFS to generate a fractal that consists of 4 pieces scaled by the factor 0.34 and one piece scaled by the factor 0.44 and rotated through the angle 2.17 radians. Your fractal should have some symmetry so that a first approximation looks like so:"
My code for this problem was:
My first iterate looked like:
and my final fractal was: